Array Assignments
Array assignments work like variable assignments. If a variable x is a reference to an array of y, then x can be a reference to z. if a variable of type z can be assigned to y.
For example, imagine that y is the AWT Component
class and z is the AWT Button class. Because a Button variable can be assigned to a Component
variable, a Button array can be assigned to a Component
array:
When an assignment like this is made, both variables button and components refer to the same heap space in memory. Changing an array element for one array element changes the element for both.
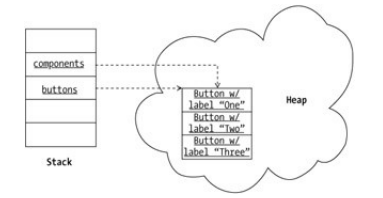
Shared memory after an array assignment
If, after assigning an array variable to a superclass array variable (as in the prior example of assigning the button array to a component array variable) you then try to place a different subclass instance into the array, an ArrayStoreException
is thrown. To continue the prior example, an ArrayStoreException
would be thrown if you tried to place a Canvas into the components array. Even though the components array is declared as an array of Component objects, because the components array specifically refers to an array of Button objects, Canvas
objects cannot be stored in the array. This is a run−time exception
as the actual assignment is legal from the perspective of a type−safe compiler
.