Initializing Arrays
When an array is first created, the runtime−environment will make sure that the array contents are automatically initialized to some known (as opposed to undefined) value. As with uninitialized instance and class variables, array contents are initialized to either the numerical equivalent of zero
, the character equivalent of \u0000
, the boolean false
, or null
for object arrays
Array Initial Values
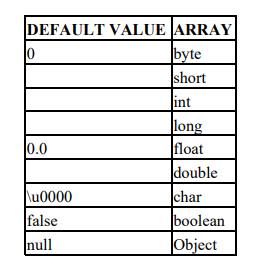
When you declare an array you can specify the initial values of the elements. This is done by providing a comma−delimited list between braces [{ }]
after an equal sign at the declaration point.
For instance, the following will create a three-element array of names:
Notice that when you provide an array initializer, you do not have to specify the length. The array length is set automatically based upon the number of elements in the comma−delimited list.
For multidimensional arrays, you would just use an extra set of parenthesis for each added dimension. For instance, the following creates a 6 × 2 array of years and events. Because the array is declared as an array of Object elements, it is necessary to use the Integer wrapper class to store each int primitive value inside. All elements within an array must be of the array's declared type, or a subclass of that type, in this case, Object, even though all the elements are subclasses
While it was easy to initialize an array when it was declared, you couldn't reinitialize the array later with a comma−delimited list unless you declared another variable to store the new array in. This is where anonymous arrays step in. With an anonymous array, you can reinitialize an array to a new set of values, or pass unnamed arrays into methods when you don't want to define a local variable to store said array
Anonymous arrays are declared similarly to regular arrays. However, instead of specifying a length within the square brackets, you place a comma−delimited list of values within braces after the brackets, as shown here:
You'll find anonymous arrays used frequently by code generators.
Passing Array Arguments and Return Values
When an array is passed as an argument to a method, a reference to the array is passed. This permits you to modify the contents of the array and have the calling routine see the changes to the array when the method returns. In addition, because a reference is passed around, you can also return arrays created within methods and not worry about the garbage collector releasing the array's memory when the method is done.