Multidimensional Arrays
Because arrays are handled through references, there is nothing that stops you from having an array element refer to another array. When one array refers to another, you get a multidimensional array. This requires an extra set of square brackets for each added dimension on the declaration line. For instance, if you wish to define a rectangular, two-dimensional array, you might use the following line:
As with one-dimensional arrays, if an array is one of primitives, you can immediately store values in it once you create the array. Just declaring it is not sufficient. For instance, the following two lines will result in a compilation-time error
because the array variable is never initialized:
If, however, you created the array between these two source line ( with something like coordinates = new int[3][4]; )
, the last line would become valid.
In the case of an array of objects, creating the multidimensional array produces an array full of null object references. You still need to create the objects to store in the arrays, too
Because each element in the outermost array of a multidimensional array is an object reference, there is nothing that requires your arrays to be rectangular (or cubic for three−dimensional arrays). Each inner array can have its own size. For instance, the following demonstrates how to create a two−dimensional array of floats where the inner arrays are sized like a set of bowling pins—the first row has one element, the second has two, the third has three, and the fourth has four:
To help visualize the final array: A triangular, bowling−pin−like array.
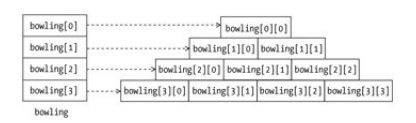
When accessing an array with multiple dimensions, each dimension expression is fully evaluated before the next dimension expression to the right is ever examined. This is important to know if an exception happens during an array access.
Keep in mind that computer memory is linear—when you access a multidimensional array you are really accessing a one−dimensional array in memory. If you can access the memory in the order it is stored, the access will be most efficient. Normally, this wouldn't matter if everything fit in memory, as computer memory is quick to hop around. However, when using large data structures, linear access performs best and avoids unnecessary swapping. In addition, you can simulate multidimensional arrays by packing them into a one−dimensional array. This is done frequently with images. The two manners of packing the one−dimensional array are row−major order, where the array is filled one row at a time; and column−major order, where columns are placed into the array
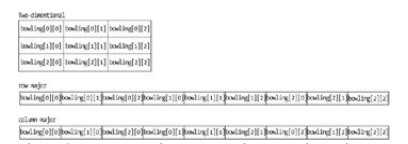
Row−major versus column−major order.